Integrate your own model
Connect an agent to your own LLM or host your own server.
Currently, the following models are natively supported and can be configured via the agent settings:
- Gemini 2.0 Flash
- Gemini 1.5 Flash
- Gemini 1.5 Pro
- Gemini 1.0 Pro
- GPT-4o Mini
- GPT-4o
- GPT-4 Turbo
- GPT-3.5 Turbo
- Claude 3.5 Sonnet
- Claude 3 Haiku
Custom LLMs let you bring your own OpenAI API key or run an entirely custom LLM server.
Overview
By default, we use our own internal credentials for popular models like OpenAI. To use a custom LLM server, it must align with the OpenAI create chat completion request/response structure.
The following guides cover both use cases:
- Bring your own OpenAI key: Use your own OpenAI API key with our platform.
- Custom LLM server: Host and connect your own LLM server implementation.
You’ll learn how to:
- Store your OpenAI API key in ElevenLabs
- host a server that replicates OpenAI’s create chat completion endpoint
- Direct ElevenLabs to your custom endpoint
- Pass extra parameters to your LLM as needed
Using your own OpenAI key
To integrate a custom OpenAI key, create a secret containing your OPENAI_API_KEY:
Custom LLM Server
To bring a custom LLM server, set up a compatible server endpoint using OpenAI’s style, specifically targeting create_chat_completion.
Here’s an example server implementation using FastAPI and OpenAI’s Python SDK:
Run this code or your own server code.
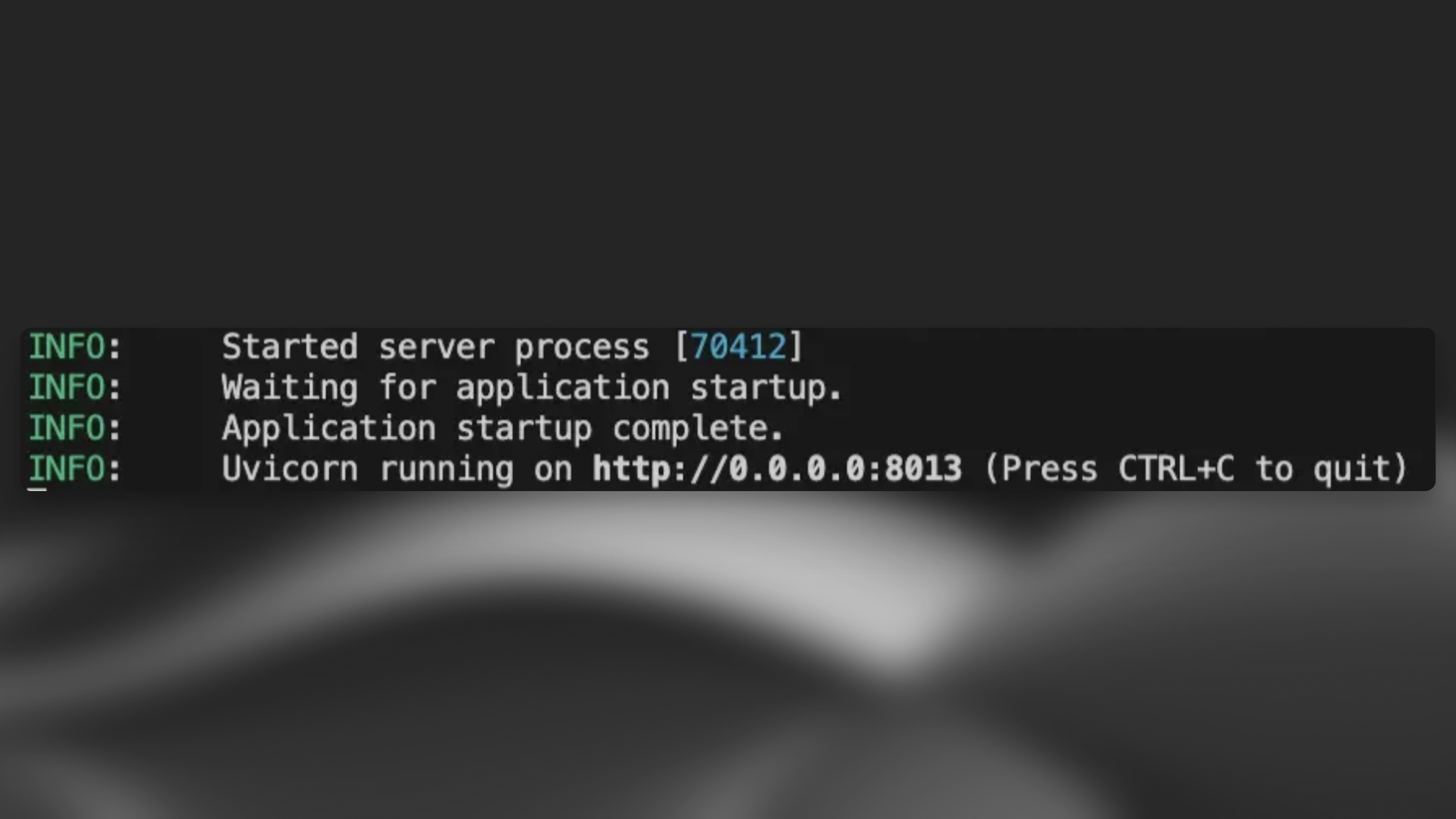
Setting Up a Public URL for Your Server
To make your server accessible, create a public URL using a tunneling tool like ngrok:
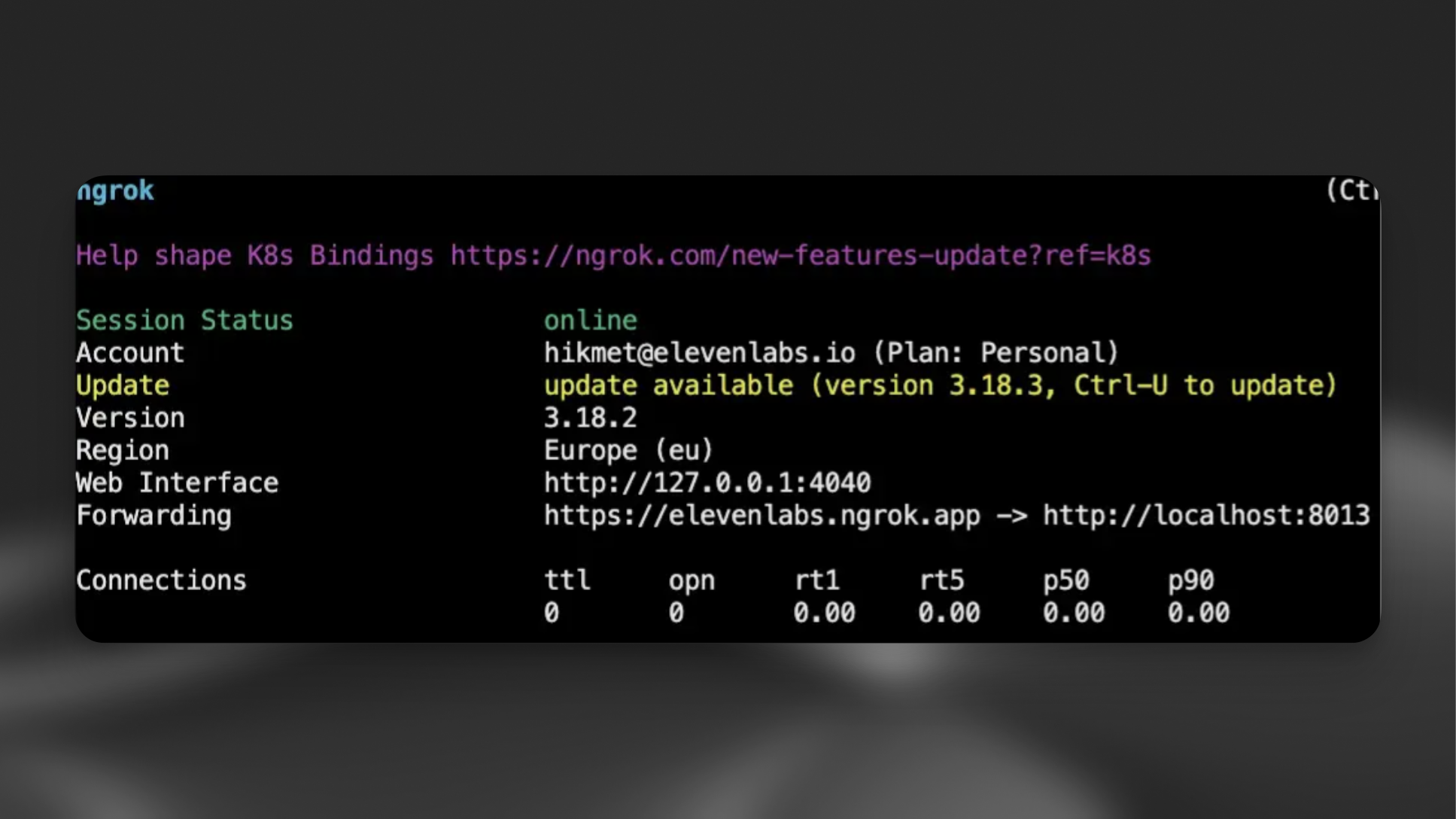
Configuring Elevenlabs CustomLLM
Now let’s make the changes in Elevenlabs
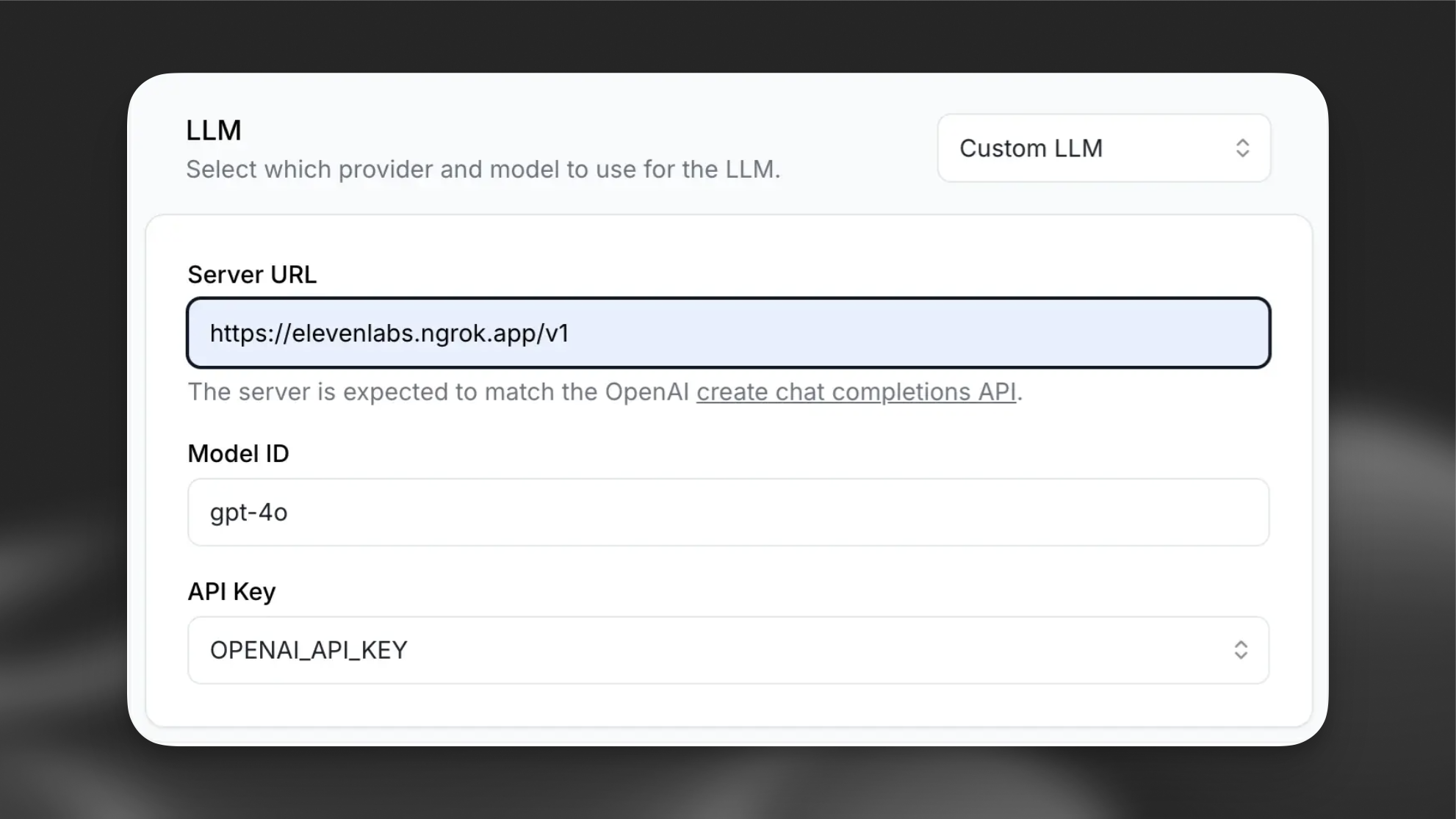
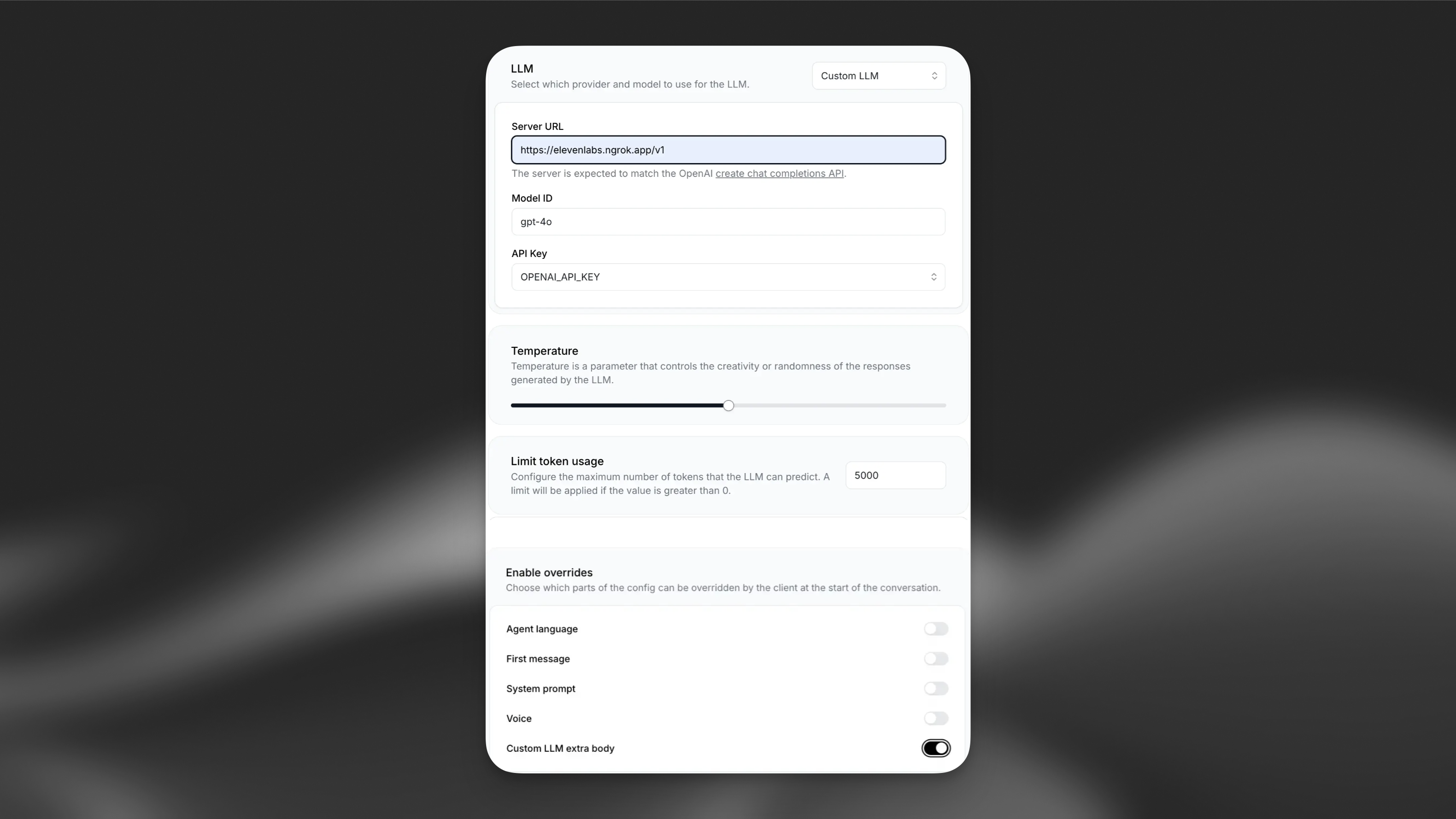
Direct your server URL to ngrok endpoint, setup “Limit token usage” to 5000 and set “Custom LLM extra body” to true.
You can start interacting with Conversational AI with your own LLM server
Additional Features
Custom LLM Parameters
You may pass additional parameters to your custom LLM implementation.