Agent transfer
Seamlessly transfer the user between Conversational AI agents based on defined conditions.
Overview
Agent-agent transfer allows a Conversational AI agent to hand off the ongoing conversation to another designated agent when specific conditions are met. This enables the creation of sophisticated, multi-layered conversational workflows where different agents handle specific tasks or levels of complexity.
For example, an initial agent (Orchestrator) could handle general inquiries and then transfer the call to a specialized agent based on the conversation’s context. Transfers can also be nested:
We recommend using the gpt-4o
or gpt-4o-mini
models when using agent-agent transfers due to better tool calling.
Enabling agent transfer
Agent transfer is configured using the transfer_to_agent
system tool.
Add the transfer tool
Enable agent transfer by selecting the transfer_to_agent
system tool in your agent’s configuration within the Agent
tab. Choose “Transfer to AI Agent” when adding a tool.
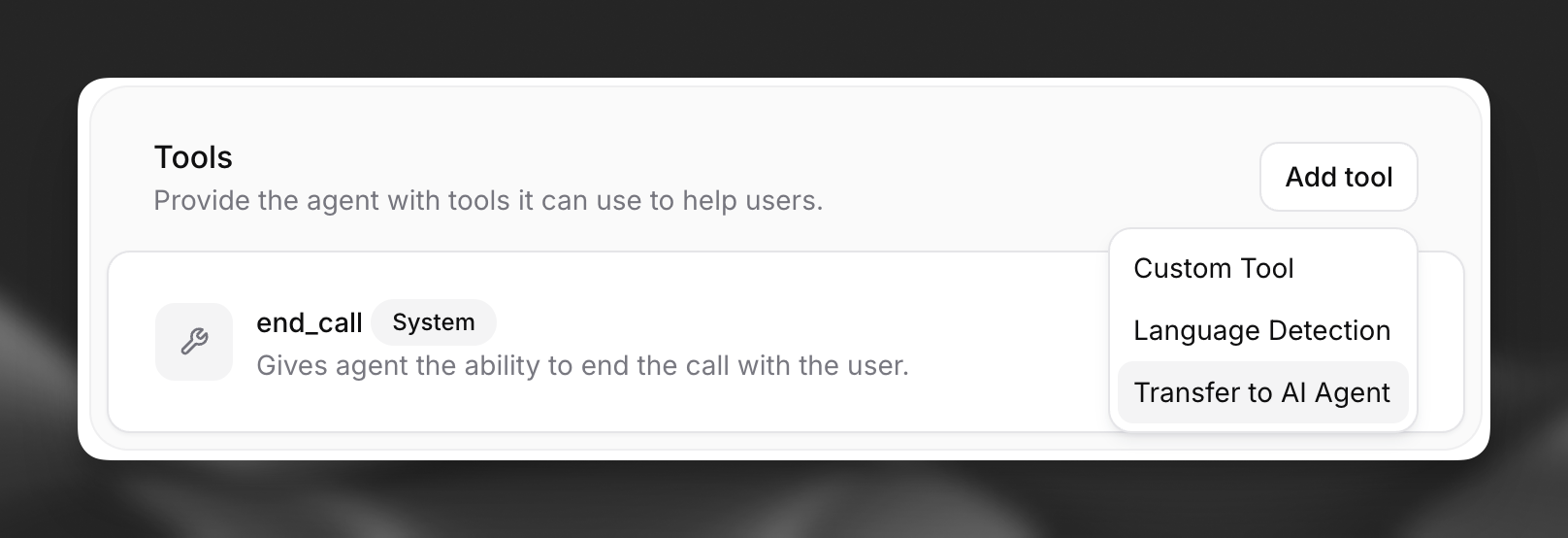
Configure tool description (optional)
You can provide a custom description to guide the LLM on when to trigger a transfer. If left blank, a default description encompassing the defined transfer rules will be used.
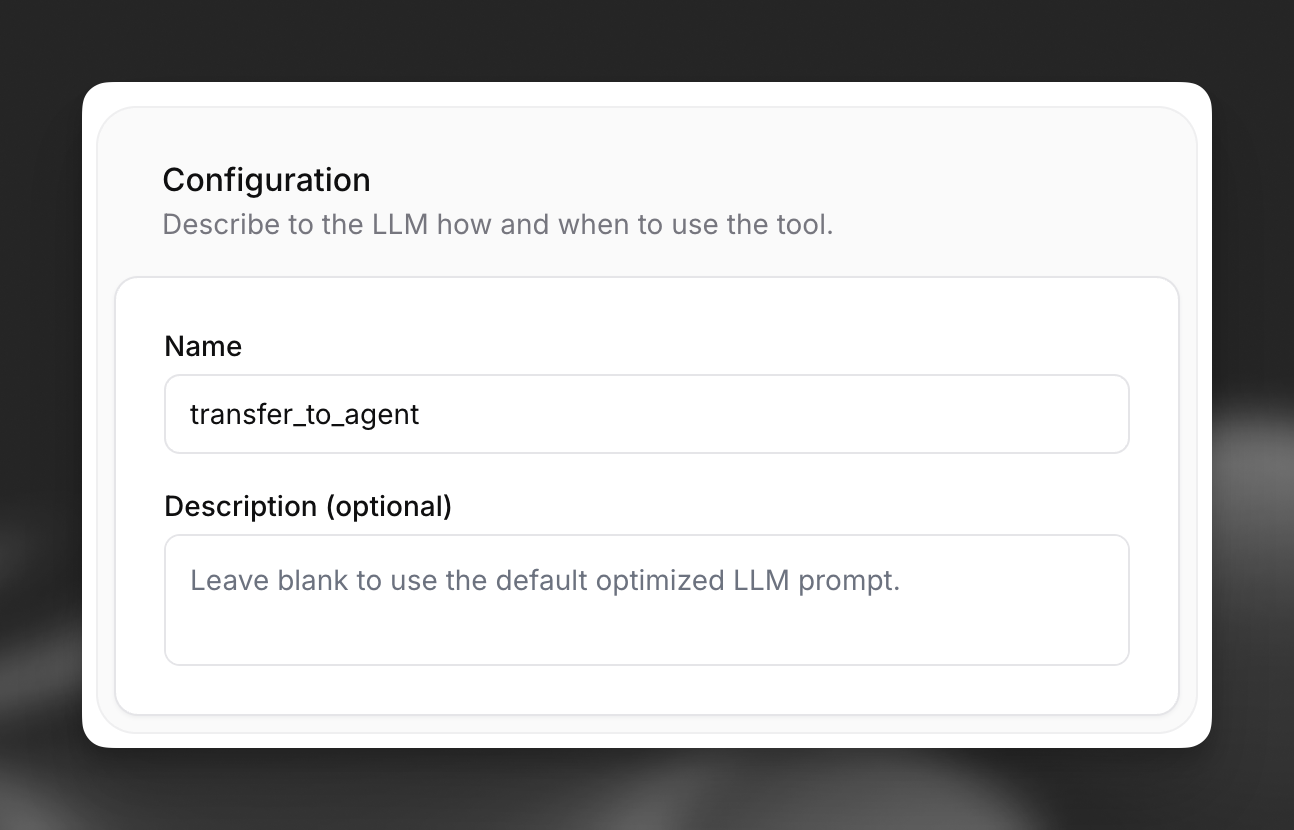
Define transfer rules
Configure the specific rules for transferring to other agents. For each rule, specify:
- Agent: The target agent to transfer the conversation to.
- Condition: A natural language description of the circumstances under which the transfer should occur (e.g., “User asks about billing details”, “User requests technical support for product X”).
The LLM will use these conditions, along with the tool description, to decide when and to which agent (by number) to transfer.
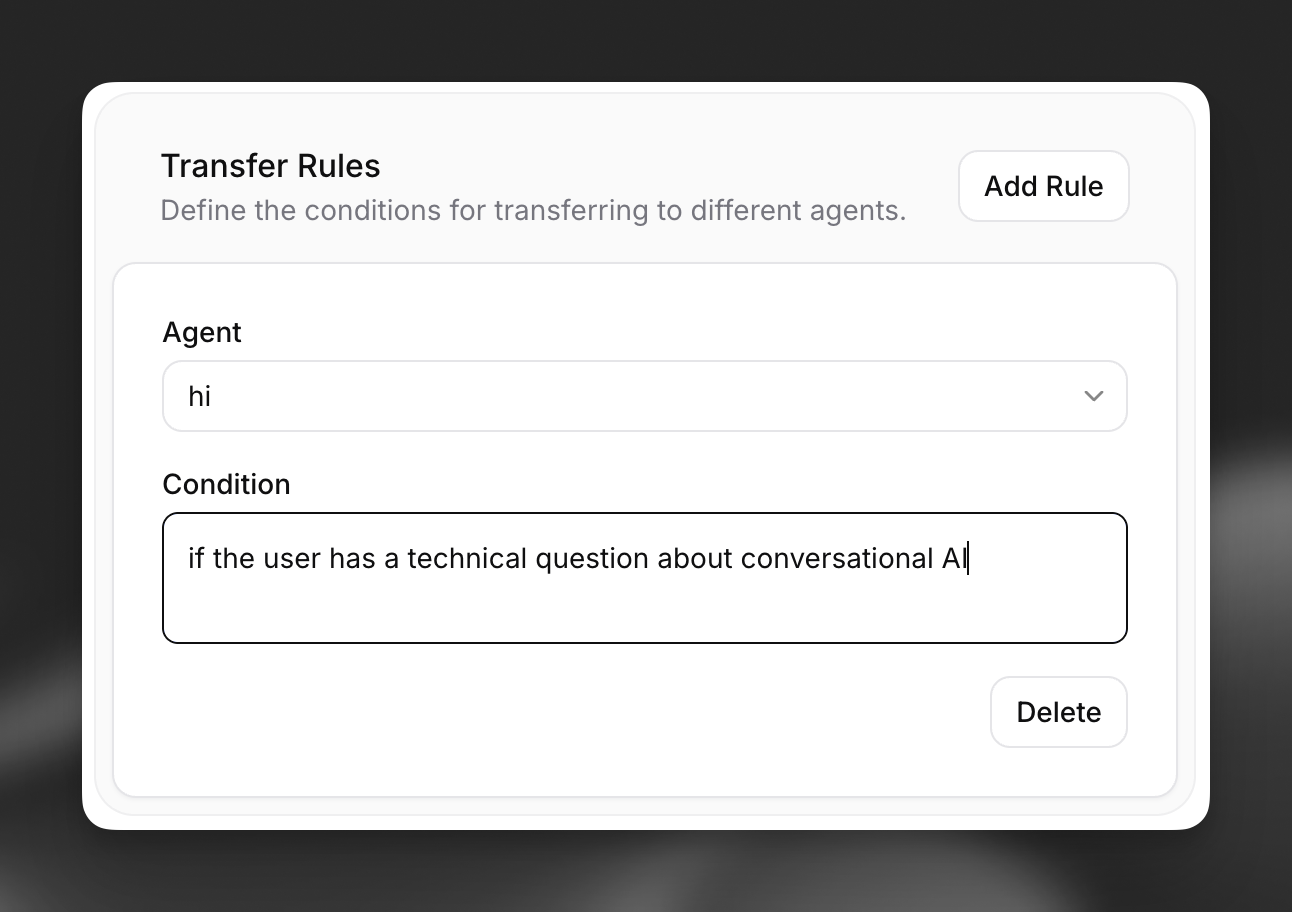
Ensure that the user account creating the agent has at least viewer permissions for any target agents specified in the transfer rules.
API Implementation
You can configure the transfer_to_agent
system tool when creating or updating an agent via the API.