Twilio custom server
Learn how to integrate a Conversational AI agent with Twilio to create seamless, human-like voice interactions.
Custom server should be used for outbound calls only. Please use our native integration for inbound Twilio calls.
Connect your ElevenLabs Conversational AI agent to phone calls and create human-like voice experiences using Twilio’s Voice API.
What You’ll Need
- An ElevenLabs account
- A configured ElevenLabs Conversational Agent (create one here)
- A Twilio account with an active phone number
- Python 3.7+ or Node.js 16+
- ngrok for local development
Agent Configuration
Before integrating with Twilio, you’ll need to configure your agent to use the correct audio format supported by Twilio.
Implementation
Javascript
Python
Looking for a complete example? Check out this Javascript implementation on GitHub.
Twilio Setup
Configure Twilio
- Go to the Twilio Console
- Navigate to
Phone Numbers
→Manage
→Active numbers
- Select your phone number
- Under “Voice Configuration”, set the webhook for incoming calls to:
https://your-ngrok-url.ngrok.app/twilio/inbound_call
- Set the HTTP method to POST
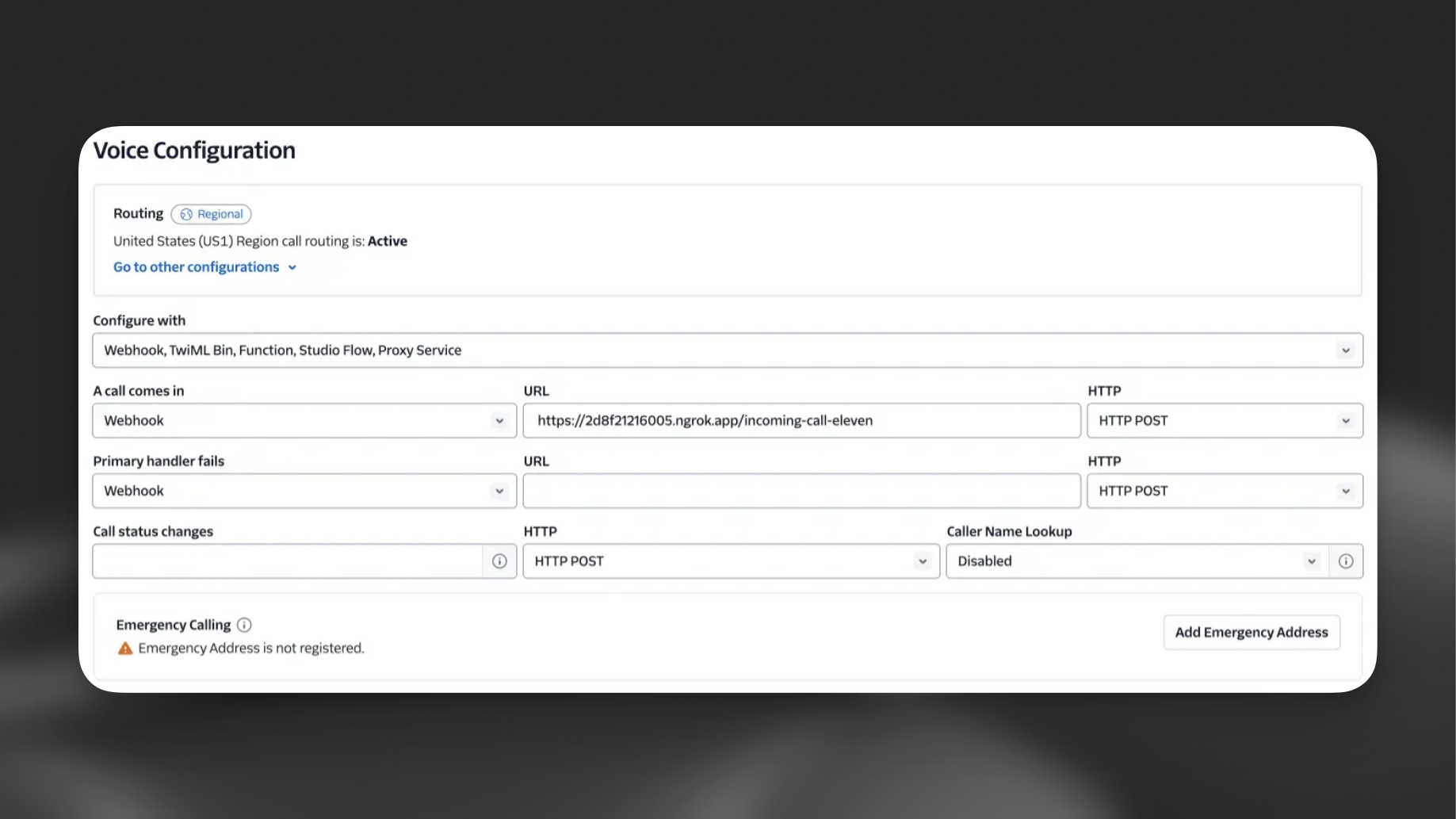
Testing
- Call your Twilio phone number.
- Start speaking - you’ll see the transcripts in the ElevenLabs console.
Troubleshooting
Connection Issues
If the WebSocket connection fails:
- Verify your ngrok URL is correct in Twilio settings
- Check that your server is running and accessible
- Ensure your firewall isn’t blocking WebSocket connections
Audio Problems
If there’s no audio output:
- Confirm your ElevenLabs API key is valid
- Verify the AGENT_ID is correct
- Check audio format settings match Twilio’s requirements (μ-law 8kHz)
Security Best Practices
Follow these security guidelines for production deployments:
- Use environment variables for sensitive information - Implement proper authentication for your endpoints - Use HTTPS for all communications - Regularly rotate API keys - Monitor usage to prevent abuse